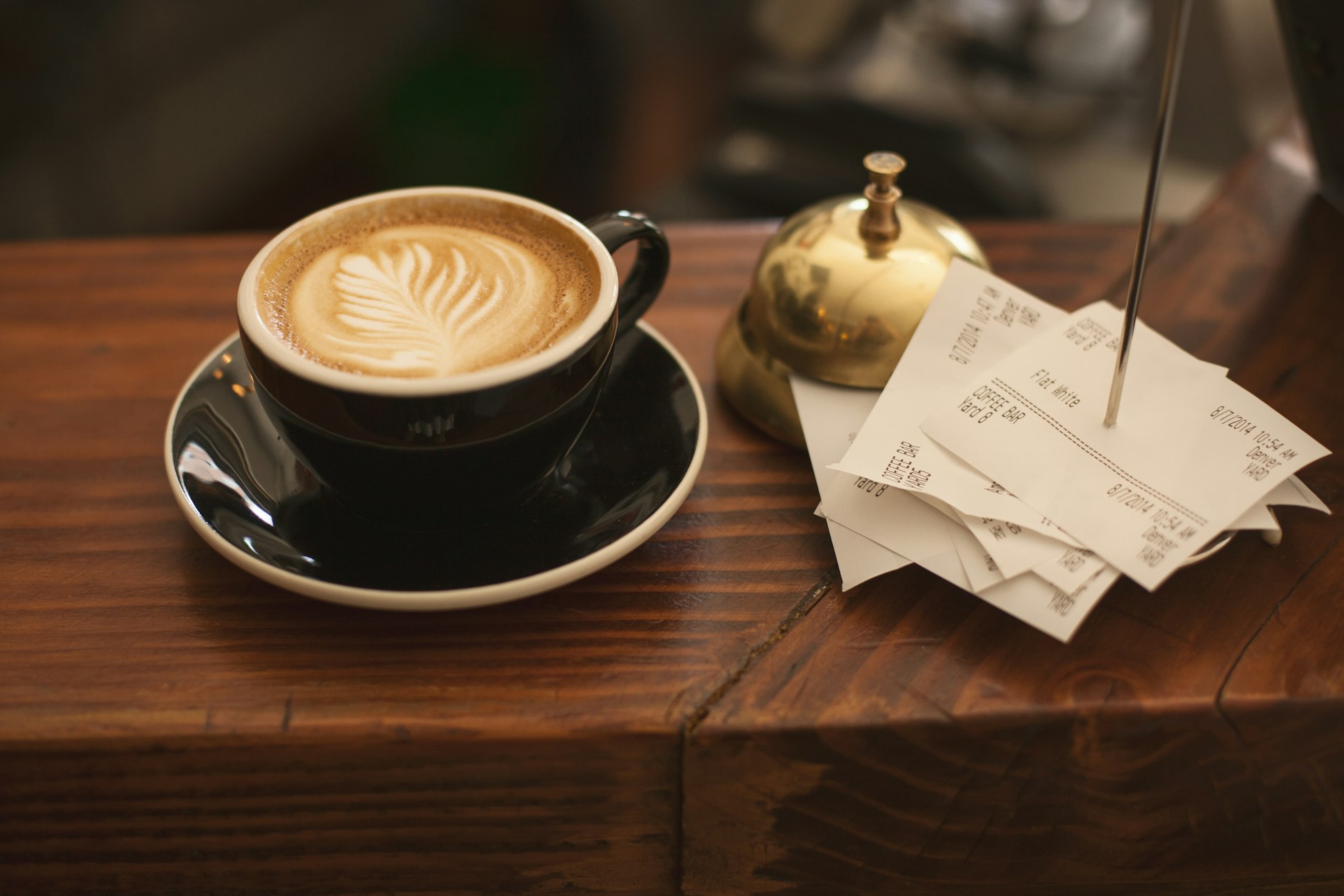
Multiple Ways to Get the First Row for Each Group in Pandas
Use case Sometimes you just want to capture the first (or last) event of something. Let’s say, you have a list of clients and want to capture their first purchase. This is useful if you want a list of new paying customers. Dataset We’re thinking about customers here, so let’s get the Online Retail Dataset from the UCI Machine Learning Repository. We can download this dataset directly using Pandas. >>> import pandas as pd >>> customers = pd....