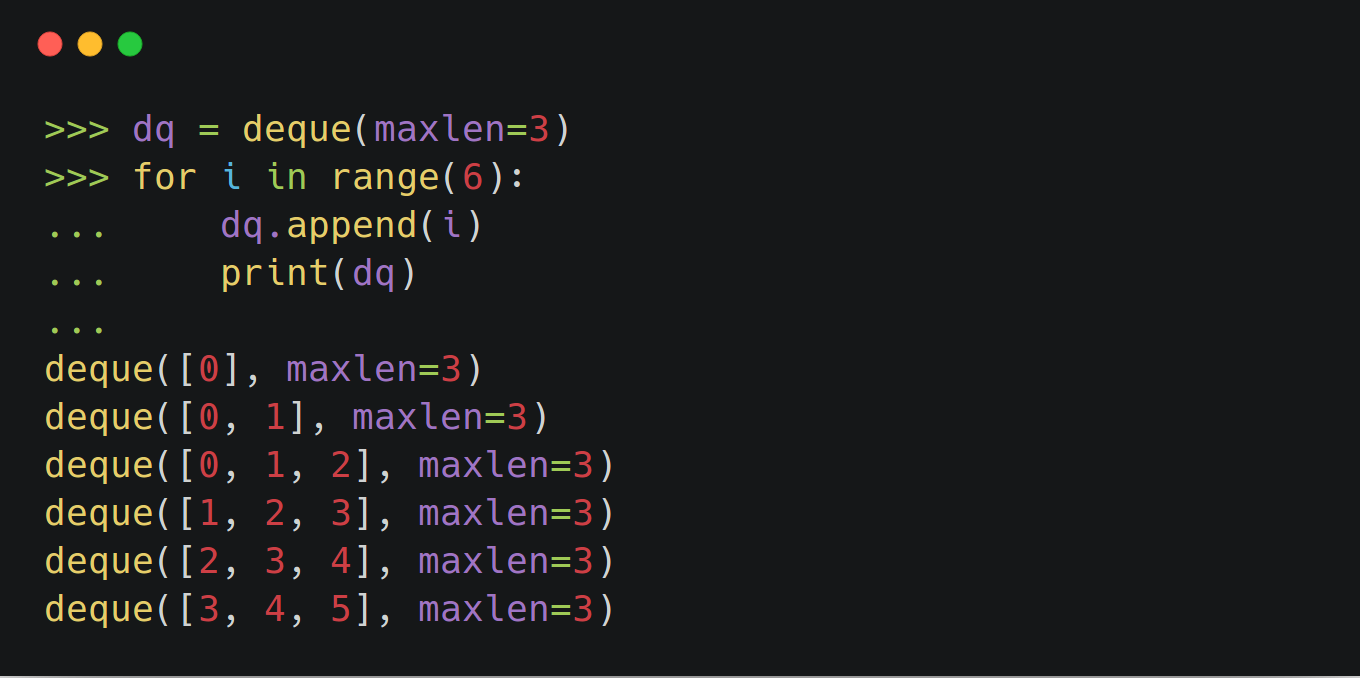
How to Handle a Deque in Python
Deques are a great way to handle memory efficient appends to a list-like object, it is a special module that allows you to handle list items in a more appropriate way. Create a deque To create a list simply import the deque module from collections library and call deque(_items_) on a variable. >>> from collections import deque >>> dq = deque('123') >>> dq deque(['1', '2', '3']) >>> type(dq) <class 'collections.deque'> Or if you wish to create an empty deque....